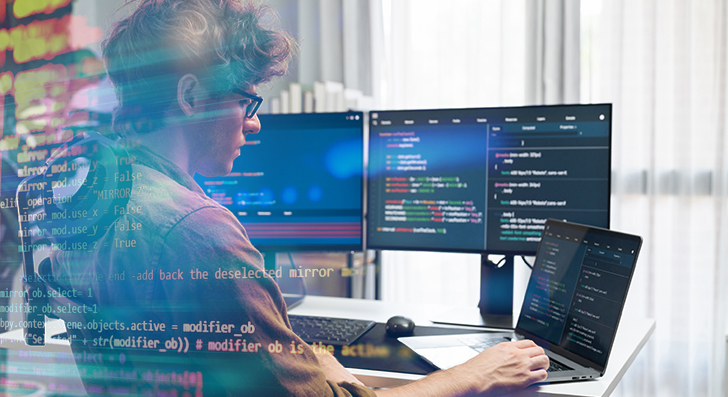
Scalability means your application can handle advancement—additional end users, more details, plus more website traffic—with no breaking. As being a developer, setting up with scalability in mind saves time and strain afterwards. Listed here’s a transparent and functional manual to help you start out by Gustavo Woltmann.
Design and style for Scalability from the beginning
Scalability isn't really one thing you bolt on afterwards—it should be section of the approach from the beginning. Lots of programs are unsuccessful after they develop speedy since the first style can’t cope with the extra load. Being a developer, you need to Consider early about how your technique will behave stressed.
Commence by coming up with your architecture to become versatile. Stay clear of monolithic codebases exactly where anything is tightly connected. As a substitute, use modular style or microservices. These designs crack your application into smaller sized, impartial sections. Each module or support can scale By itself with out impacting The full procedure.
Also, consider your database from day just one. Will it have to have to handle 1,000,000 buyers or just a hundred? Choose the proper form—relational or NoSQL—dependant on how your data will develop. Program for sharding, indexing, and backups early, Even when you don’t have to have them yet.
An additional crucial position is to stop hardcoding assumptions. Don’t produce code that only is effective less than present-day situations. Give thought to what would materialize In case your user base doubled tomorrow. Would your app crash? Would the database decelerate?
Use design designs that guidance scaling, like concept queues or celebration-pushed programs. These support your application take care of far more requests devoid of obtaining overloaded.
Whenever you Construct with scalability in mind, you're not just getting ready for achievement—you are minimizing potential head aches. A perfectly-prepared technique is simpler to keep up, adapt, and develop. It’s better to arrange early than to rebuild later on.
Use the correct Database
Choosing the correct databases is usually a essential Portion of developing scalable purposes. Not all databases are crafted the exact same, and using the Incorrect one can slow you down or maybe lead to failures as your app grows.
Start out by comprehension your information. Can it be hugely structured, like rows inside a desk? If Of course, a relational database like PostgreSQL or MySQL is a superb in shape. They're strong with associations, transactions, and consistency. In addition they help scaling procedures like read through replicas, indexing, and partitioning to deal with extra targeted traffic and information.
If the info is more versatile—like user action logs, item catalogs, or files—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are superior at handling huge volumes of unstructured or semi-structured details and may scale horizontally additional effortlessly.
Also, look at your study and produce designs. Are you executing lots of reads with less writes? Use caching and skim replicas. Have you been managing a heavy compose load? Take a look at databases that may handle substantial produce throughput, or perhaps function-dependent data storage methods like Apache Kafka (for short term facts streams).
It’s also smart to Feel in advance. You may not want State-of-the-art scaling features now, but selecting a databases that supports them suggests you won’t require to change later on.
Use indexing to hurry up queries. Steer clear of needless joins. Normalize or denormalize your knowledge determined by your obtain styles. And always check database efficiency when you mature.
In short, the best database is determined by your app’s framework, pace demands, And just how you be expecting it to improve. Choose time to select correctly—it’ll help you save loads of trouble afterwards.
Improve Code and Queries
Speedy code is essential to scalability. As your application grows, each individual compact hold off provides up. Improperly created code or unoptimized queries can slow down performance and overload your procedure. That’s why it’s imperative that you Establish successful logic from the start.
Begin by writing clean up, uncomplicated code. Prevent repeating logic and remove just about anything unwanted. Don’t select the most advanced Resolution if an easy 1 works. Keep the capabilities quick, focused, and straightforward to check. Use profiling instruments to seek out bottlenecks—spots in which your code requires much too very long to run or uses far too much memory.
Following, take a look at your database queries. These normally sluggish matters down a lot more than the code itself. Be sure Each and every question only asks for the data you truly require. Stay clear of Pick *, which fetches all the things, and in its place pick unique fields. Use indexes to speed up lookups. And prevent performing too many joins, Primarily across massive tables.
If you recognize a similar information being requested time and again, use caching. Store the outcomes briefly applying resources like Redis or Memcached and that means you don’t really have to repeat costly operations.
Also, batch your database functions after you can. As an alternative to updating a row one after the other, update them in teams. This cuts down on overhead and makes your app a lot more successful.
Make sure to exam with big datasets. Code and queries that perform wonderful with a hundred documents might crash whenever they have to manage one million.
To put it briefly, scalable applications are speedy applications. Keep your code tight, your queries lean, and use caching when needed. These actions aid your application remain easy and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to manage additional buyers plus more traffic. If everything goes through 1 server, it'll rapidly become a bottleneck. That’s where load balancing and caching are available in. These two tools assistance keep the application rapidly, steady, and scalable.
Load balancing spreads incoming site visitors across multiple servers. Instead of a person server accomplishing the many get the job done, the load balancer routes users to distinctive servers dependant on availability. What this means is no solitary server gets overloaded. If a person server goes down, the load balancer can send out traffic to the others. Applications like Nginx, HAProxy, or cloud-dependent answers from AWS and Google Cloud make this easy to arrange.
Caching is about storing knowledge temporarily so it may be reused quickly. When people request the same information and facts once again—like a product site or even a profile—you don’t need to fetch it with the database when. It is possible to serve it from the cache.
There are 2 typical different types of caching:
1. Server-facet caching (like Redis or Memcached) retailers data in memory for rapidly access.
2. Shopper-aspect caching (like browser caching or CDN caching) suppliers static information near the user.
Caching lessens database load, increases speed, and would make your app far more efficient.
Use caching for things that don’t improve usually. And normally ensure your cache is current when information does adjust.
In short, load balancing and caching are basic but potent instruments. Together, they help your application tackle much more end users, continue to be quick, and Get well from complications. If you plan to increase, you would like both.
Use Cloud and Container Equipment
To develop scalable applications, you may need applications that let your app expand quickly. That’s where by cloud platforms and containers come in. They give you versatility, lessen set up time, and make scaling A great deal smoother.
Cloud platforms like Amazon Web Solutions (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and products and services as you need them. You don’t need to purchase hardware or guess potential capability. When targeted traffic boosts, you may insert extra means with just some clicks or quickly applying vehicle-scaling. When targeted visitors drops, you could scale down to economize.
These platforms also offer services like managed databases, storage, load balancing, and security tools. You can focus on setting up your application in place of controlling infrastructure.
Containers are Yet another crucial Instrument. A container offers your application and almost everything it has to run—code, libraries, configurations—into just one device. This causes it to be straightforward to move your application amongst environments, out of your laptop to your cloud, with no surprises. Docker is the most popular tool for this.
Once your app uses various containers, instruments like Kubernetes enable you to manage them. Kubernetes handles deployment, scaling, and Restoration. If a single portion of your application crashes, it restarts it instantly.
Containers also make it very easy to independent aspects of your app into services. You may update or scale components independently, which happens to be great for performance and dependability.
In short, employing cloud and container resources suggests you are able to scale speedy, deploy quickly, and recover promptly when issues transpire. If you would like your application to grow with no restrictions, begin working with these tools early. They preserve time, cut down threat, and make it easier to stay focused on making, not fixing.
Check Anything
Should you don’t watch your software, you won’t know when items go Completely wrong. Checking assists you see how your application is accomplishing, spot concerns early, and make greater conclusions as your application grows. It’s a key Portion of making scalable units.
Start by tracking simple metrics like CPU utilization, memory, disk House, and response time. These tell you how your servers and providers are undertaking. Instruments like Prometheus, Grafana, Datadog, or New Relic will let you obtain and visualize this data.
Don’t just keep track of your servers—check your app also. Keep watch over just how long it requires for end users to load web pages, how frequently problems come about, and wherever they come about. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring within your code.
Arrange alerts for vital complications. Such as, In the event your reaction time goes earlier mentioned a Restrict or even a support goes down, you ought to get notified instantly. This assists you fix issues speedy, generally in advance of end users even recognize.
Monitoring is usually handy if you make adjustments. In the event you deploy a fresh function and find out a spike in glitches or slowdowns, you'll be able to roll it back right before it brings about actual damage.
As your application grows, site visitors and information maximize. With no monitoring, you’ll miss out on signs of hassle right up until it’s as well late. But with the ideal equipment in place, you keep in control.
Briefly, monitoring allows you maintain your app trusted and scalable. It’s not nearly recognizing failures—it’s about comprehending your procedure and ensuring it really works nicely, even stressed.
Last Feelings
Scalability isn’t just for massive companies. Even modest apps want a solid foundation. more info By coming up with cautiously, optimizing correctly, and utilizing the proper instruments, you are able to Make applications that expand effortlessly with no breaking stressed. Begin modest, Imagine large, and Make smart.